How to integrate FilePond with your Basin forms
Tom Fast on Jun 6 2023
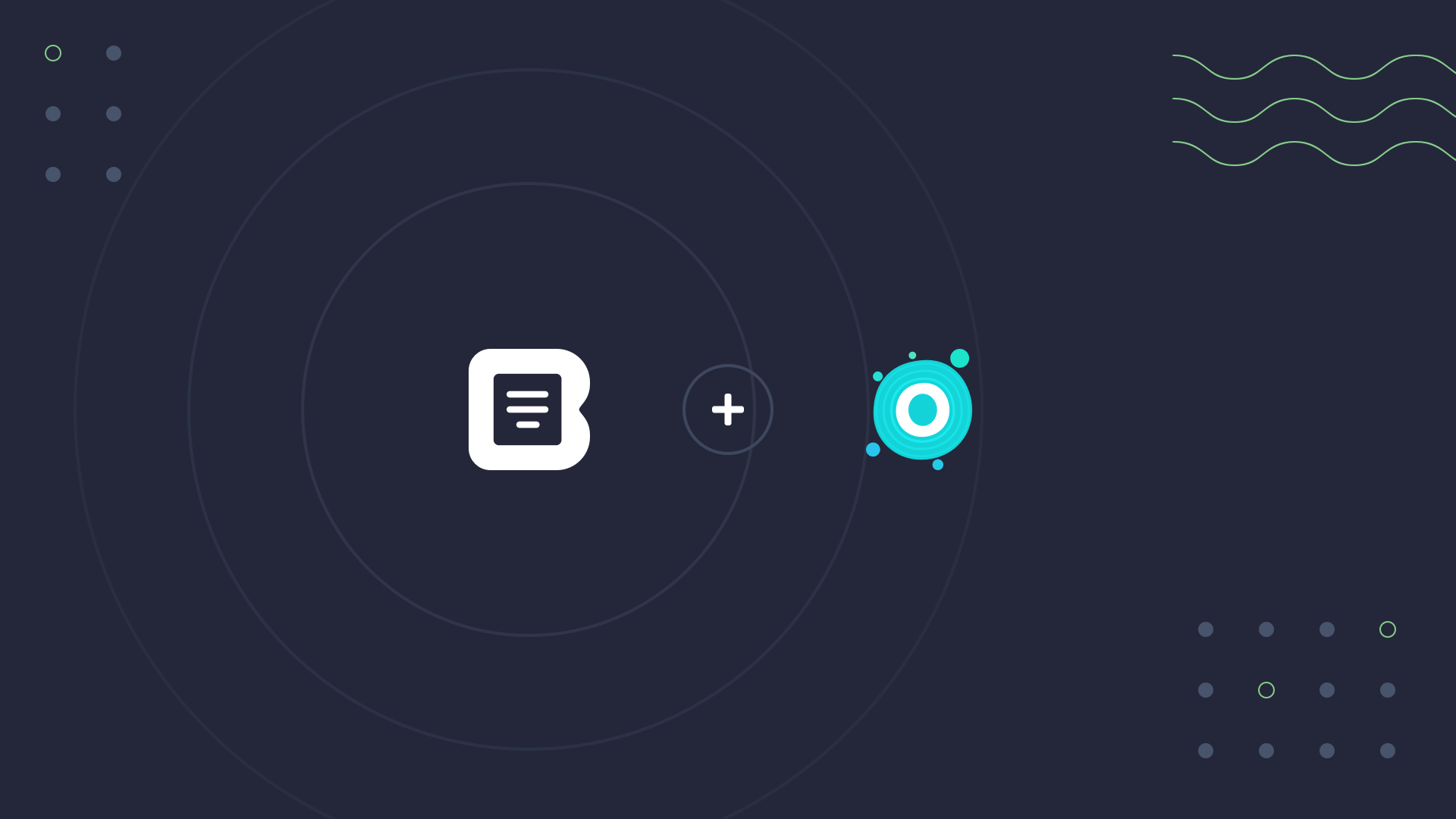
Here's a quick guide that demonstrates how to utilize FilePond, a versatile JavaScript library, to process file uploads with Basin's form endpoints.
Basin offers a plethora of features including persistent submissions storage, advanced spam features, email configurations and more. Pairing it with FilePond, you can manage file uploads with ease and elegance. Let's dive in.
Getting started
Before we begin, ensure that you've got the necessary components:
- FilePond Library
- Basin Account
<!-- Load FilePond library -->
<link href="https://unpkg.com/filepond/dist/filepond.css" rel="stylesheet">
<link href="https://unpkg.com/filepond-plugin-image-preview/dist/filepond-plugin-image-preview.css" rel="stylesheet">
Next, prepare a form on your page with a file input and a Basin endpoint. Here's an example:
<form accept-charset="UTF-8" action="[your_form_endpoint]" method="POST" id="basinUploadForm" class="flow" enctype="multipart/form-data">
<!-- Your form fields go here -->
<input type="file" name="attachments[]" multiple> <input id="submitButton" class="button button--primary" type="submit" value="Submit"> </form>
Ensure that you replace "[your_form_endpoint]" with your unique Basin form endpoint.
Next, we need to initialize FilePond and tie it to our input. This will provide us with a beautiful, customizable, and accessible file upload experience:
<script src="https://unpkg.com/filepond-plugin-image-preview/dist/filepond-plugin-image-preview.js"></script>
<script src="https://unpkg.com/filepond/dist/filepond.js"></script>
<script>
// Register the plugin
FilePond.registerPlugin(FilePondPluginImagePreview);
const inputElement = document.querySelector('input[type="file"]');
const formElement = document.querySelector('#basinUploadForm');
const submitButton = document.querySelector('#submitButton');
// Create the FilePond instance
const pond = FilePond.create(inputElement, {
instantUpload: false
});
</script>
Lastly, let's handle form submission and file uploading. We use the Fetch API to post the form to Basin and handle the responses:
formElement.addEventListener('submit', (e) => {<
e.preventDefault();
// Disable the submit button and change its text to "Uploading..."
submitButton.disabled = true;
submitButton.value = "Uploading...";
const formData = new FormData(formElement);
pond.getFiles().forEach((fileItem) => {
formData.append('attachments[]', fileItem.file);
});
fetch(formElement.action, {
method: formElement.method,
body: formData,
redirect: 'manual' // Prevent automatic redirection
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
// Redirect to the specified URL after successful upload
window.location.href = response.headers.get('Location') || 'https://usebasin.com/thank-you';
})
.catch(error => {
console.error('Error:', error);
// Enable the submit button and change its text back to "Submit" if there's an error
submitButton.disabled = false;
submitButton.value = "Submit";
});
});
</script>
Make sure you replace 'https://usebasin.com/thank-you' with your custom redirect URL if you have one. This will act as a fallback in case the Location is not found in the response header.
And there you have it! You are now able to forward your form submissions to Basin with FilePond for file handling. This grants you a robust file upload experience, easy spam filtering, secure submission storage, and more.
For any assistance with integrating FilePond with Basin, don't hesitate to get in touch with our support team. We're always eager to help you out.
Get Started with a Free Basin account today!
Discover the benefits of Basin and how it can help streamline your forms. Sign up now and explore our features.
Sign Up for Free