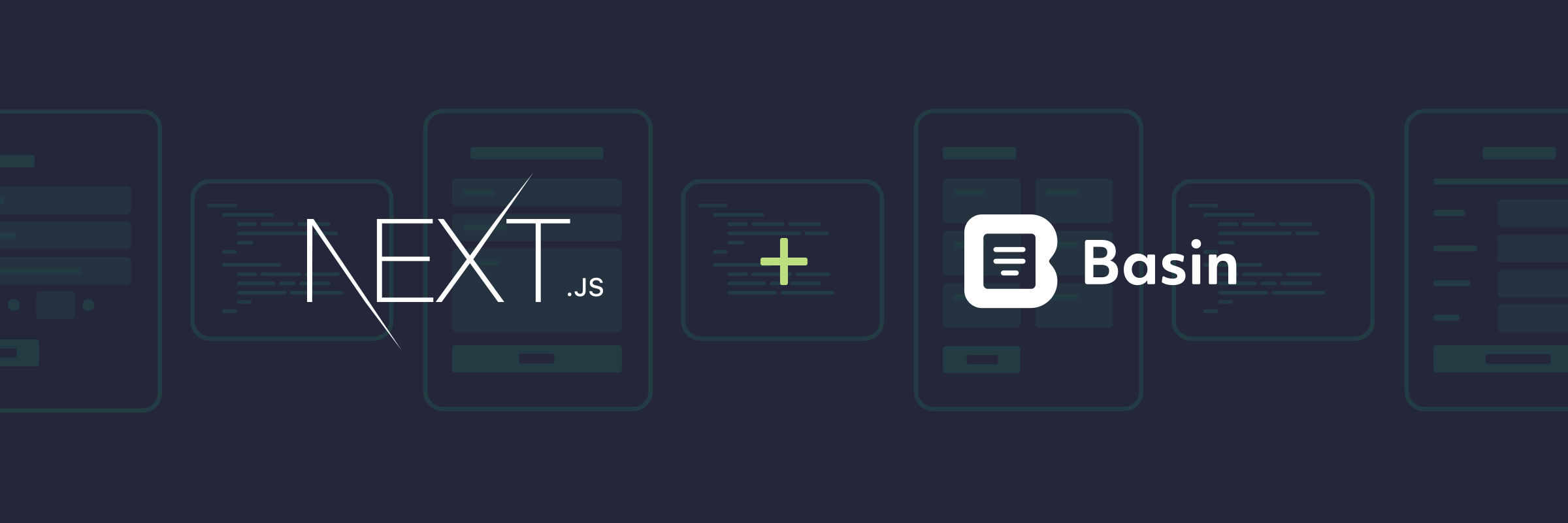
Build React forms with NextJS
In this guide, we'll show you how to quickly add a contact form to your NextJS project and connect it to Basin.
Basin is a no code form backend that pairs nicely with static site generators, jamstack tools and frontend development frameworks like NextJS.
NextJS is a popular React based frontend development framework built by Vercel. It's great for creating lightning fast, search engine friendly jamstack websites and applications that are scalable and easy to maintain.
By following this guide, you'll have a working contact form on your NextJS static website or app that sends you email notifications when people submit.
Requirements
You'll need a Basin account, which you can create for free here, and an existing web project built with NextJS. If you don't already have an existing project, we'll go through the process of creating a sample project and contact form in the steps that follow.
If you’re not familiar with NextJS or how it works, be sure to check out the official documentation to learn more before getting started.
Step 1 - Create a new NextJS project
Let's setup a project by following the steps in the NextJS Getting Started documentation. In your terminal, run the follow command to create a new site:
npx create-next-app@latest
The CLI will prompt you with a few questions to configure the desired project information and setup. Once everything is installed you will see a terminal message with instructions for navigating to your site and running it locally.
Go to the new directory for the new website:
cd your-folder-name
Start the web server locally:
npm run develop
Navigate to http://localhost:3000 in your browser and you should now see the sample site with the following screen:
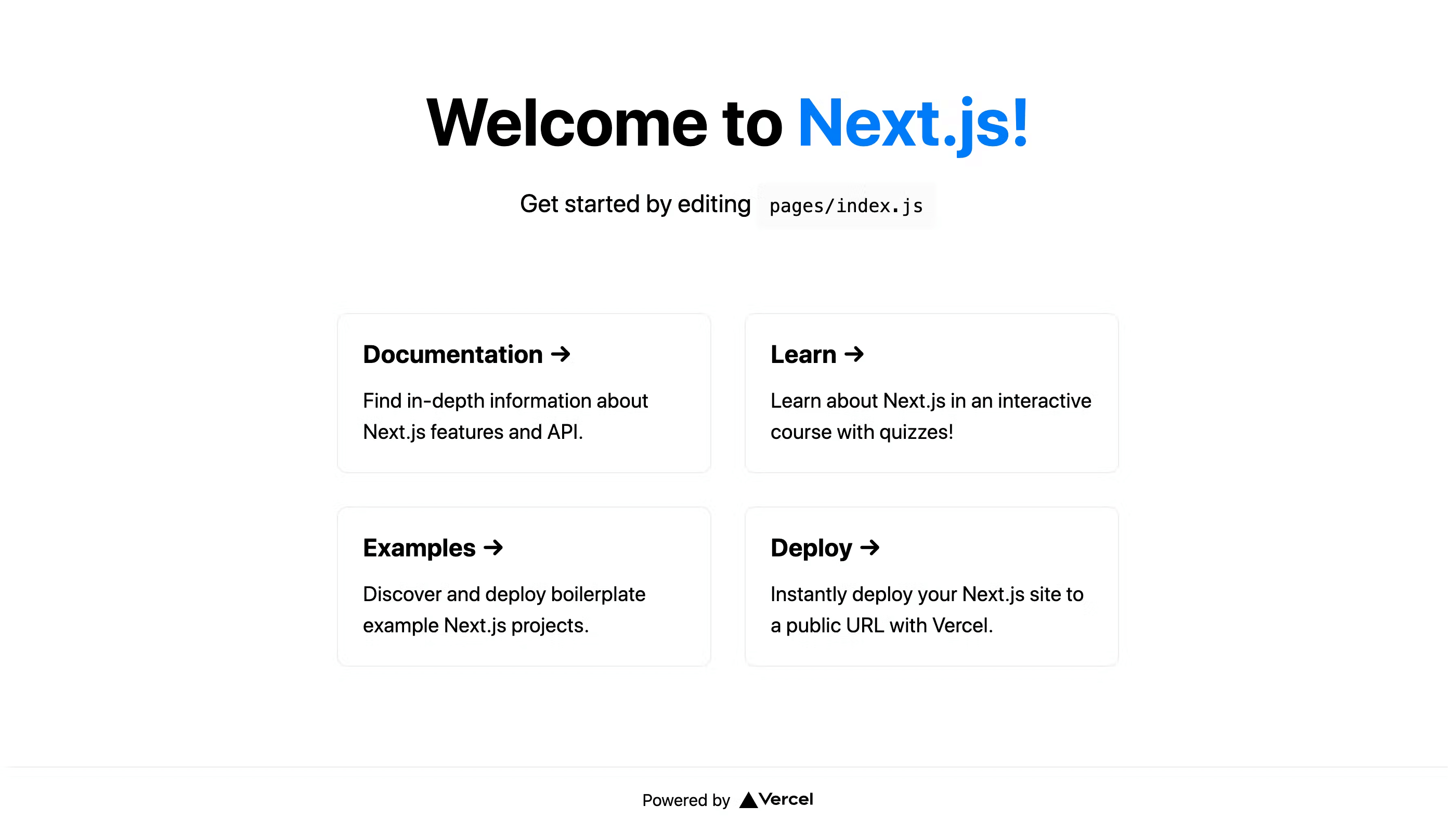
Nicely done! You just created a new NextJS site. Next, we'll look at how to add a basic contact form to the project.
The Downsides of Building Forms within NextJS
Although Next.js provides a way for you to handle form data within the framework, there are multiple reasons why going the "build your own" route may not be the best choice.
- Extra overhead and configuration - In order to process forms in Next.js, you will need to create an API endpoint where you will send the form data. This requires setting up a form handler that will process and receive requests from the client and send back a response as JSON. Although this can lend itself to a custom experience, the extra boilerplate and configuration needed to set this up properly can be tedious and time consuming.
- Added complexity - Simple forms with a small number of inputs may not be too verbose to handle. However, as soon the amount of inputs your form processes continues to grow, the logic to handle customized forms can grow exponentially. This can lead to unexpected bugs and complicated logic within the code.
- No integration support for third party applications - Most custom built form handling doesn't support integration with other external tools, which limits your ability to pass submission data on to other applications for workflow automation.
Create Better NextJS Forms with Basin
Basin makes it easy to bypass the limitations of creating forms within the NextJS framework. We built it with the needs of Jamstack developers in mind, and it helps you and your site visitors get the most out of the form submission experience. Here are a few reasons why Basin is a great form backend for NextJS projects.
- Simplified setup - Basin takes the complexity out of form building. Instead of adding a bunch of logic to handle submission data, you simply setup the form to your liking, pass the form endpoint as the value for the `action` field and, Basin handles the rest. No complicated logic is required.
- Inuitive and customizable - Basin's intuitive dashboard gives you complete control of the form building and frontend user experience. You can easily build and configure specific form elements and styles to suit your needs.
- Endless integration possibilities - Basin makes form data processing and management work more seamless by giving you the tools to send your form data to popular third-party services. We have built-in support Zapier, Webhooks, and Slack integrations, and we’ll be adding support for more native integrations in the future.
Now that you're aware of some of potential pitfalls of building your own form handler within NextJS, let's take a look at how simple this process is within Basin.
Step 2 - Create a Form Endpoint in Basin
After logging in to your Basin account, you can create a new form endpoint from the Forms index page. Simply fill out the fields and click “Create Form”. You'll use this same process for every form endpoint you create.
After your new form endpoing is created, it will appear in the My Forms list. Select it to view the Submissions page, where you can see the inbox, check form performance analytics, and access additional features like integrations, mailer settings, etc.
You'll also notice a form endpoint URL located on the right side of the screen under the sub-navigation. Copy this URL, as you will use it in the next step to link Basin to the new contact form in your NextJS project.
Step 3 - Create and Connect your React Form
With your Basin form endpoint all ready to go, its time to build your form. Since NextJS uses React, you can simply build a form component that can be added to any page in your project. Create a new file called contact.js
in the src/pages
directory and add the following code block in the file:
import * as React from “react”;
const Contact = () => {
return (
<main>
<form action=“https://usebasin.com/f/<YOUR_FORM_ID>” method=“POST”>
<label htmlFor=“name”>Name </label>
<input type=“text” name=“name” id=“name” required />
<label htmlFor=“email”>Email </label>
<input type=“email” name=“email” id=“email” required />
<button type=“submit”>Submit</button>
</form>
</main>
);
};
export default Contact;
Paste the form endpoint URL you copied from Basin into your form’s [action]
attribute as shown in the code block above. In order for your form to submit to Basin, the <YOUR_FORM_ID>
in your form code needs to match the ID of the endpoint you created in the previous step.
In NextJS, any React component defined in the pages
directory automatically becomes a page, so you can navigate to http://localhost:3000/contact to view the form in the browser.
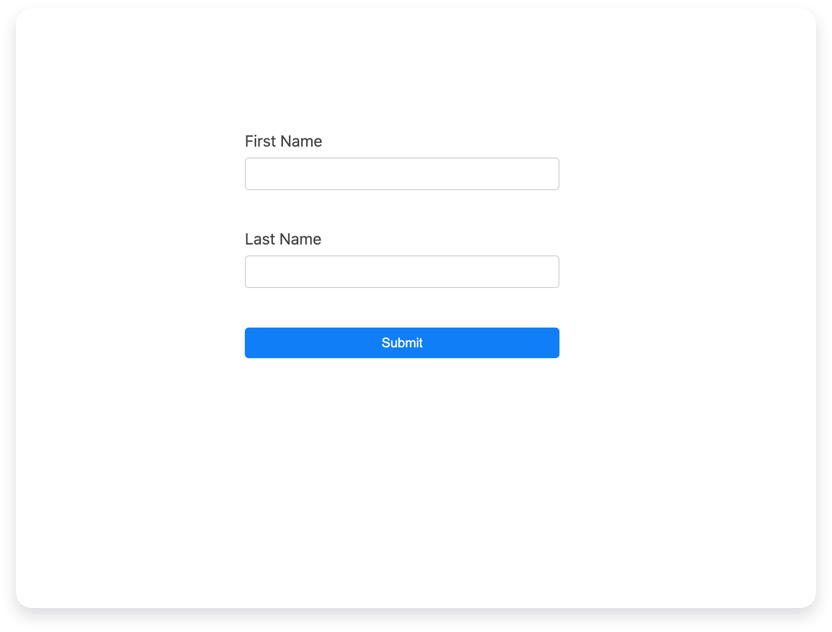
And that's it! Now after filling out and submitting the form you should see the default submission success page displayed.
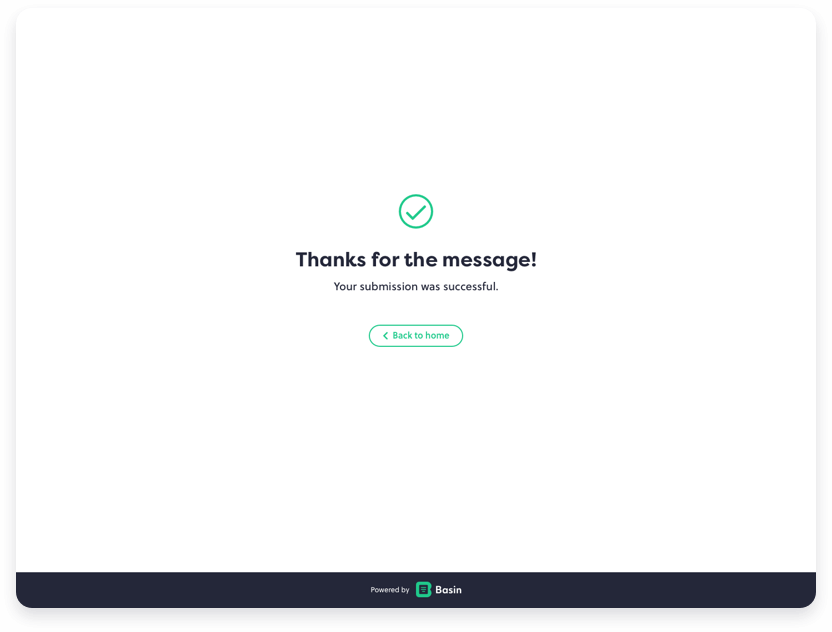
In addition, after submitting you should see a new message in the forms inbox when viewing your form's Submissions tab in Basin.
Congrats! Basin is now powering the contact form on your NextJS site!
Bonus — Spam Prevention with Honeypot
The "honeypot" technique can be used to add an additional layer of protection as it attempts to lure bots into completing a field that human users can't detect. By including a hidden honeypot field in your form for spam bots to fill out, the submission will be ignored when a value is entered and submitted.
A custom honeypot field name can be specified within your form's Spam Settings in Basin. The code example below shows how you can add the default honeypot field to a form in React:
<form action=“https://usebasin.com/f/<YOUR_FORM_ID>” method=“POST”>
…
<input type="hidden" name="_gotcha" />
…
</form>
The hidden honeypot field _gotcha
can be used in conjunction with other spam filtering methods we support, like Google reCPATCHA, hCAPTCHA, and domain restrictions, and using a custom field name makes it work even better.
Check out our Plans and Pricing for a full breakdown of features to see how Basin can add value to your workflow and grow with your needs.
Conclusion
In this guide, you learned how quick and easy it is to get a Basin form backend up and running on your NextJS site. To learn how Basin works with other jamstack frameworks, static site generators, and CMS platforms, check out our other step-by-step installation guides.
Thanks for reading, and happy form building!
Have questions or need help with something specific? Read the docs or get in touch.