Building User-Friendly HTML Contact Forms: A Beginner's Guide
Anthony Penner on Jan 26 2024
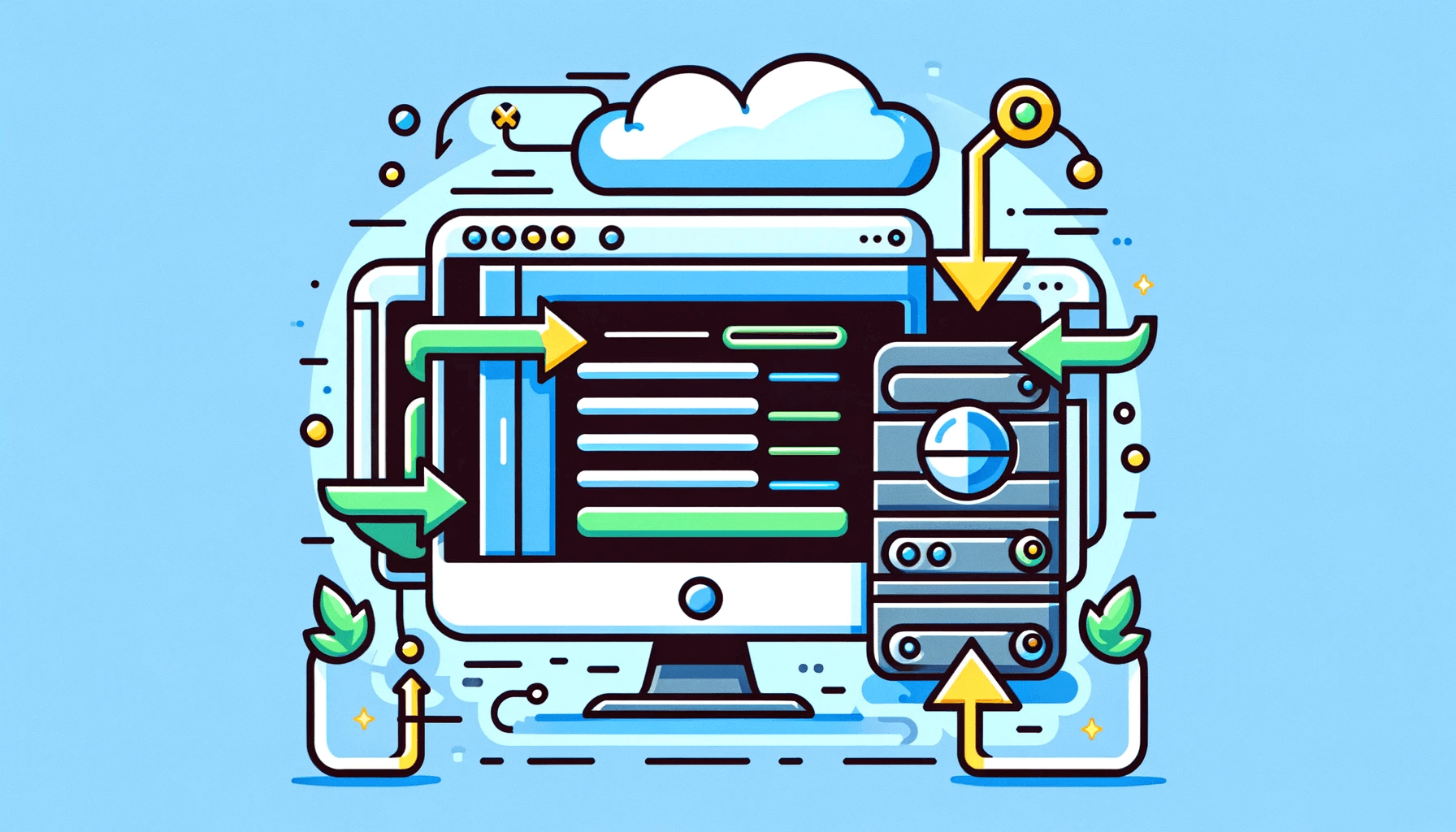
Introduction
Creating a contact form for your website might seem a bit daunting at first, especially if you're new to web development. But don't worry, it's actually quite straightforward! In this guide, we'll walk you through the basics of building a simple HTML contact form. You don't need to be a tech wizard – with just a few lines of code, you'll have a working form ready for your site.
Along the way, we'll mention tools like Basin, which can help handle form submissions, but the focus here is on the HTML part. By the end of this guide, you'll have a clear understanding of how to create a contact form that your website visitors can use to get in touch with you.
Ready to dive in? Let's get started with the basics!
The Basics of HTML Contact Forms
Creating an HTML contact form is like putting together a simple puzzle. You just need a few pieces, and once you put them in the right place, you've got a form that lets people send you messages through your website. Let's look at these pieces:
The Basic Structure
Here's a basic example of what an HTML contact form looks like:
<form action="where-your-form-goes" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name"><br><br> <label for="email">Email:</label> <input type="email" id="email" name="email"><br><br> <input type="submit" value="Send"> </form>
In this example, we have:
- A form tag that wraps everything up. It's like a container for your form.
- Input fields for the name and email. This is where people will type their info.
- A submit button. When clicked, it sends the form's data somewhere.
Making It Work
The `action` attribute in the form tag tells the form where to send the data when the submit button is pressed. If you're using a service like Basin, this is where you'll put the link they give you. If not, it will be a link to a script on your server that processes the form data.
Adding a Bit of Style
You can make your form look nicer with a bit of CSS. Here’s a simple example:
form { margin: 20px; } input[type=text], input[type=email] { width: 100%; padding: 12px; margin: 8px 0; display: inline-block; border: 1px solid #ccc; box-sizing: border-box; } input[type=submit] { background-color: #4CAF50; color: white; padding: 14px 20px; margin: 8px 0; border: none; cursor: pointer; width: 100%; }
See the Pen Basic HTML Form Example by Anthony Penner (@anthonypenner) on CodePen.
Enhancing Your Contact Form
Once you've got the basics down, you can add more elements to your HTML contact form to make it even better. Let's explore some common form elements that can enhance functionality and user experience.
Text Areas
For longer messages, a text area is perfect. Here’s how to add one:
<label for="message">Message:</label>
<textarea id="message" name="message" rows="4" cols="50">
</textarea>
Radio Buttons and Checkboxes
<p>How did you hear about us?</p>
<div>
<input type="radio" id="search" name="source" value="search">
<label for="search">Search Engine</label><br>
<input type="radio" id="social" name="source" value="social">
<label for="social">Social Media</label><br>
<input type="radio" id="friend" name="source" value="friend">
<label for="friend">Friend</label>
</div>
<p>What services are you interested in?</p>
<div>
<input type="checkbox" id="design" name="service" value="design">
<label for="design">Design</label><br>
<input type="checkbox" id="dev" name="service" value="dev">
<label for="dev">Development</label><br>
<input type="checkbox" id="seo" name="service" value="seo">
<label for="seo">SEO</label>
</div>
Dropdown Menus
<label for="country">Country:</label> <select id="country" name="country"> <option value="usa">United States</option> <option value="uk">United Kingdom</option> <option value="canada">Canada</option> <!-- Add more options here --> </select>
Enhancing User Experience
Form Submission Handling
The Journey of Form Data
- User Submits the Form: When the submit button is clicked, the browser collects all the data entered into the form.
- Data Sent to the Server: The form data is sent to the server specified in the action attribute of the <form> tag. If you're using a third-party service like Basin, you'll put their URL here. Otherwise, it'll be a script on your server.
- Server Processes the Data: Once the server receives the data, it's processed according to your script or the service you're using. This could involve storing it in a database, sending an email, or other actions.
- Response to the User: After processing, the server might redirect the user to a new page, such as a thank-you page, or display a message confirming that their submission was successful.
A Simple PHP Example
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
// Process the data...
// For example, send an email or store it in a database
// Redirect or display a message
echo "Thank you for your submission!";
}
?>
Security and Validation
Best Practices for Contact Form Design
Clarity is Key
- Label Clearly: Each field in your form should have a clear label so users know exactly what information to enter.
- Keep It Simple: Only ask for the information you really need. Too many fields can overwhelm users and reduce the chances of them completing the form.
Make It Responsive
- Mobile-Friendly Design: With more people using smartphones, your form should look good and work well on all devices.
- Test on Different Screens: Check how your form appears on different screen sizes to ensure a consistent experience.
User-Friendly Features
- Easy Navigation: Users should be able to tab through fields easily.
- Input Validation: Help users correct their data before submitting the form. For example, if an email address is not in the correct format, let them know.
Accessible Design
- Keyboard Accessibility: Ensure users can navigate your form using a keyboard.
- Screen Reader Friendly: Use proper HTML elements and attributes so screen readers can interpret your form correctly.
Aesthetically Pleasing
- Consistent Design: Your form should match the overall design of your website.
- Pleasing Visuals: Use colors and fonts that are easy on the eyes and align with your brand.
Integrating Basin with Your HTML Contact Form
Simple Integration
<form action="https://usebasin.com/f/your-unique-endpoint" method="post">
<!-- Your form fields go here -->
</form>
Why Choose Basin?
- Spam Checking: Basin automatically filters out spam, keeping your inbox clean.
- Easy Email Handling: Set up automatic email responses to your form submissions.
- Custom Email Templates: Personalize the emails sent from your form with custom templates.
- Powerful Integrations: Connect your form to other tools and services effortlessly.
- Form Builder: If you don’t want to write HTML, use Basin's form builder to create forms visually.
- Basin JS: Enhance your forms with Basin's JavaScript library for more functionality.
See the Pen Basin Form Action: Kitchen Sink Example by Anthony Penner (@anthonypenner) on CodePen.
Conclusion
Get Started with a Free Basin account today!
Discover the benefits of Basin and how it can help streamline your forms. Sign up now and explore our features.
Sign Up for Free